I am maintaining a WordPress website for my department. As a part of that, sometimes I need to add custom code into the webpages to automize some work. At the beginning, it was not too hard. First, I finish all the code locally with a single HTML document and make sure it works properly. Second, I copy all the code in <style>
, <body>
, and <script>
tags, and pasted them into a WordPress Custom HTML block. It works pretty well, just like the native WordPress element.
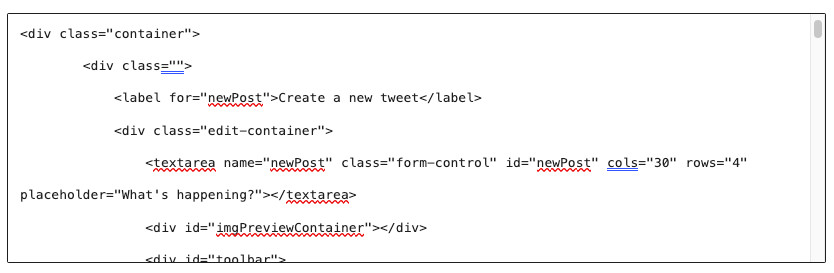
The Goal
I have two goals in this project.
- The embedded iframe should look native.
- I can edit all the source code with a native code editor and sync the page content with GitHub.
- It can be reused easily in WordPress.
Solutions
Give the embedded iframe a native look.
The following two functions were inspired by the solution I found on Stackoverflow. The original answer was a little bit more complex, which considered compatibility for the old browsers. The basic idea is to set the iframe height to the height of the source web page when loaded. To get that height, we can send the height from the source to the iframe. Following two functions are used:
// The function that send the height of the source page content. function sendHeightForIframe(mainContainer){ parent.postMessage(document.querySelector(mainContainer).offsetHeight, '*'); } // The function that receives the height from source page and set the height for the iframe. function getHeightForIframe(trackedIframe) { // Listen for a message from the iframe. window.addEventListener("message", function (e) { if (isNaN(e.data)) return; // replace #iTweeContainer with what ever what ever iframe id you need const trackedElement = document.querySelector(trackedIframe); trackedElement.style.height = e.data + 'px'; trackedElement.style.width = "1px"; trackedElement.style.minWidth = "100%"; }, false); }
Here is the usage of two functions. First, add the function sendHeightForIframe
to the page to be embeded. I added the selector “body” to the parameter.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Embeded Page</title> </head> <body id="contentBody"> <div> This is the page to be embeded. </div> <script> sendHeightForIframe("body"); function sendHeightForIframe(mainContainer){ parent.postMessage(document.querySelector(mainContainer).offsetHeight + 50, '*'); } </script> </body> </html>
Then, I added the iframe to the target page, call the function getHeightForIframe
and pass the id of the iframe.
<iframe src="url_of_the_embeded_page" id="trackedIframe" frameborder="0" width="100%" style="min-height: 100vh"></iframe> <script type="text/javascript"> getHeightForIframe("#trackedIframe"); function getHeightForIframe(trackedIframe) { // Listen for a message from the iframe. window.addEventListener("message", function (e) { if (isNaN(e.data)) return; // replace #iTweeContainer with what ever what ever iframe id you need const trackedElement = document.querySelector(trackedIframe); trackedElement.style.height = e.data + 'px'; trackedElement.style.width = "1px"; trackedElement.style.minWidth = "100%"; }, false); } </script>
Host the source code on GitHub
I wrote the entire project in VS code and hosted it on GitHub. To make it visible, I created published the project with GitHub page. However, you can host it anywhere you want.
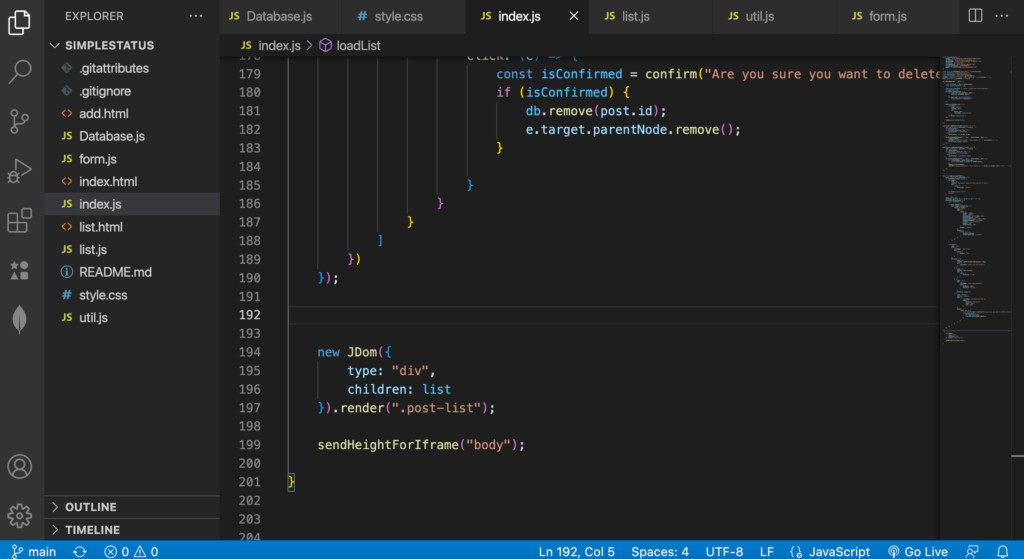
Reuse the code with short coder
Next, you can add the iframe code and the script to a HTML block of the WordPress page and it will work well. However, it would be inconvenient to edit and reuse. A better approach is to attach the code somewhere and insert a shortcode into the page. To achieve that, I installed a plugin called “Shortcoder.” I create a shortcode in this plugin and pasted the code.
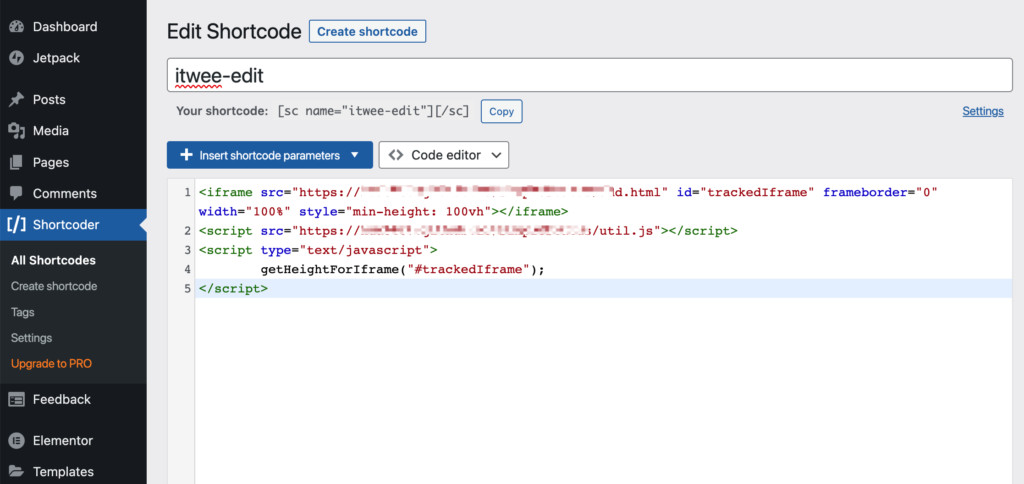
On the target WordPress page, I inserted the shortcode.

In this way, I can reuse the code by adding the shortcode on any page. More importantly, I can update the code with a native editor like Visual Studio Code without logging in my WordPress dashboard.